Finding Models
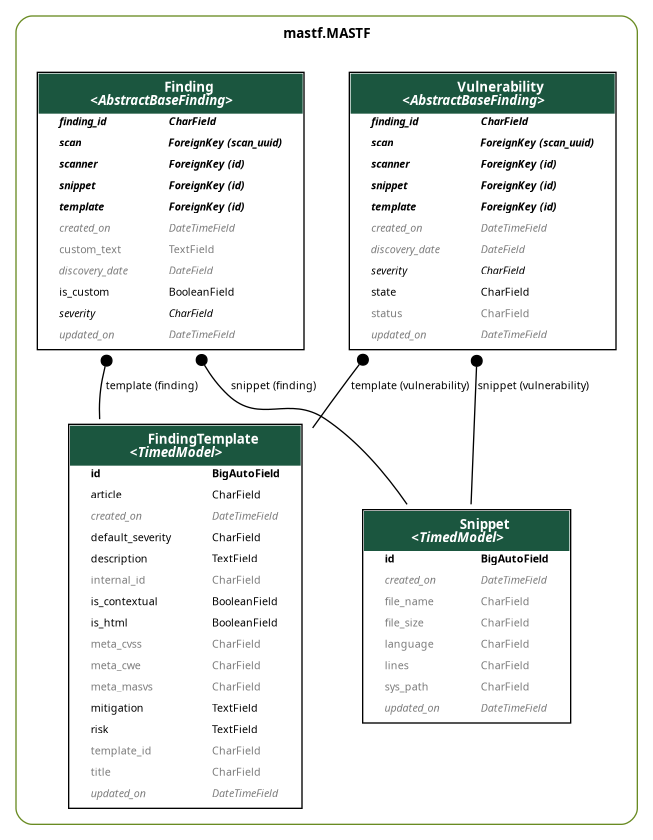
Figure 1: This view tries to illustrate the connections and relationships between models of this page.
- class mastf.MASTF.models.Snippet(*args, **kwargs)[source]
Django model for storing code snippets with optional information.
- file_name
Stores the name of the file where the snippet was found (optional).
- file_size
Stores the size of the file where the snippet was found (optional).
- language
Specifies the programming language this finding was found in (optional)
- lines
Stores lines that should be highlighted.
- sys_path
Stores the path to the file where the snippet was found (optional, internal).
- class mastf.MASTF.models.FindingTemplate(*args, **kwargs)[source]
Represents a model for storing information about a finding template. This model is used to create, update and delete templates which then are used to generate findings or vulnerabilities. Each template is assigned a unique ID and an internal ID that is used as a key for finding the template in the database.
Examples:
Creating a new finding template:
>>> template = FindingTemplate( template_id="1", internal_id="example-template", title="Example Template", description="This is an example template.", default_severity=Severity.HIGH, risk="High", mitigation="Apply the recommended security patches.", article="https://example.com/article" ) >>> template.save()
Updating an existing finding template:
>>> template = FindingTemplate.objects.get(internal_id="example-template") >>> template.title = "Updated Example Template" >>> template.description = "This is an updated example template." >>> template.save()
Or deleting an existing finding template:
>>> template = FindingTemplate.objects.get(internal_id="template-1") >>> template.delete()
- article
A link to an article associated with the finding.
- default_severity
The default severity assigned to the finding. See
Severity
for more information.
- description
A description of the template.
- internal_id
A unique internal ID assigned to the template used as a key for finding the template in the database.
- is_contextual
Tells analyzer tasks to use custom generated text when creating new Finding objects.
- is_html
Indicates whether the text of this finding has html format.
- static make_internal_id(title: str) str [source]
Generate an internal ID for the template based on its title.
Returns a string in the format
<title>
with spaces, colons, and underscores replaced with hyphens and consecutive hyphens removed. The resulting string is then converted to lowercase. This method is used to- Parameters:
title (str) – the template’s title
- Returns:
the internal id
- Return type:
str
- static make_uuid(*args) str [source]
Used to generate a unique ID for the template.
- Returns:
Returns a unique UUID string in the format
FT-{uuid4()}-{uuid4()}
.- Return type:
str
- meta_cvss
Default CVSS score associated with this finding.
- meta_cwe
CWE identifier assigned to this template.
- meta_masvs
Additional reference to the mobile verification standard.
- mitigation
A description of the mitigation steps that can be taken to resolve the finding.
- risk
A description of the risk associated with the finding.
- template_id
A unique ID assigned to the template.
- title
The title of the template.
- class mastf.MASTF.models.AbstractBaseFinding(*args, **kwargs)[source]
AbstractBaseFinding is an abstract model that serves as a base class for all findings in the vulnerability scanner.
- discovery_date
Stores the date this vulnerability or finding was detected.
- finding_id
A unique identifier for the finding
- scan
A foreign key that links the finding to a specific scan.
- scanner
The scanner that found the vulnerability or finding
- severity
The severity of the finding
- snippet
A foreign key that links the finding to a specific code snippet
- static stats(model, member: User = None, project: Project = None, scan: Scan = None, team: Team = None, base=None, bundle: Bundle = None) namespace [source]
This static method returns a namespace with statistics about the number of findings of a specific model and severity for a specific member, project, scan, team, base or bundle.
- Parameters:
model (
django.db.models.Model
) – the model to get the statistics for (either Vulnerability or Finding)member (
User
, optional) – the member to filter the statistics for (defaults to None).project (
Project
, optional) – the project to filter the statistics for (defaults to None).scan (
Scan
, optional) – the scan to filter the statistics for (defaults to None).team (
Team
, optional) – the team to filter the statistics for (defaults to None).base (
django.db.models.QuerySet
, optional) – the base queryset to filter the statistics for (defaults to None).bundle (
Bundle
, optional) – the bundle to filter the statistics for (defaults to None).
- Returns:
a namespace containing the count, high, critical, medium, low, and other statistics for the given parameters.
- Return type:
The method works by filtering the given model based on the parameters passed and then returning a namespace containing the statistics of the filtered query set. If no parameter is passed, the method returns an empty namespace.
The statistics returned are:
count
- the total number of findingshigh
- the number of findings with severity HIGHcritical
- the number of findings with severity CRITICALmedium
- the number of findings with severity MEDIUMlow
- the number of findings with severity LOWother
- the number of findings that do not belong to any of the severity levels mentioned aboverel_count
- the relative count, which is the count if count is greater than 0, and 1 otherwise.
Usage example:
To get the count of findings of the AbstractBaseFinding model for a specific project, you could use the following code:
project = Project.objects.get(pk=1) count = AbstractBaseFinding.stats(model=Finding, project=project)
- template
The finding template used to create the finding
- class mastf.MASTF.models.Finding(*args, **kwargs)[source]
A model that represents a finding.
Inherits from AbstractBaseFinding model and adds some additional attributes.
- custom_text
Stores custom text for a finding.
- discovery_date
Stores the date this vulnerability or finding was detected.
- finding_id
A unique identifier for the finding
- is_custom
A boolean indicating if the finding is custom or not.
- static make_uuid(*args) str [source]
A static method that generates a unique ID for a finding.
- Returns:
A string representing the unique ID.
- Return type:
str
- scan
A foreign key that links the finding to a specific scan.
- scanner
The scanner that found the vulnerability or finding
- severity
The severity of the finding
- snippet
A foreign key that links the finding to a specific code snippet
- template
The finding template used to create the finding
- class mastf.MASTF.models.Vulnerability(*args, **kwargs)[source]
A model that represents a vulnerability.
Inherits from AbstractBaseFinding model and adds some additional attributes.
- discovery_date
Stores the date this vulnerability or finding was detected.
- finding_id
A unique identifier for the finding
- static make_uuid(*args) str [source]
A static method that generates a unique ID for a vulnerability.
- Returns:
A string representing the unique ID.
- Return type:
str
- scan
A foreign key that links the finding to a specific scan.
- scanner
The scanner that found the vulnerability or finding
- severity
The severity of the finding
- snippet
A foreign key that links the finding to a specific code snippet
- state
Stores the state of a vulnerability. See
State
for information about the current state of a vulnerability.
- status
The status of this vulnerability
- template
The finding template used to create the finding