Core Models
Before we introduce the model classes being used the most in this project, there is another utility class that comes with some special behaviour:
- class mastf.MASTF.models.namespace(**kwargs)[source]
Simple class that stores its attributes in a separate dict.
It behaves like a normal dictionary with variable assignment possible. So, for example:
>>> var = namespace(foo="bar") >>> var.foo 'bar'
Variables can still be defined after the object has been created:
>>> var = namespace() >>> var.bar = 2 >>> var {"bar": 2}
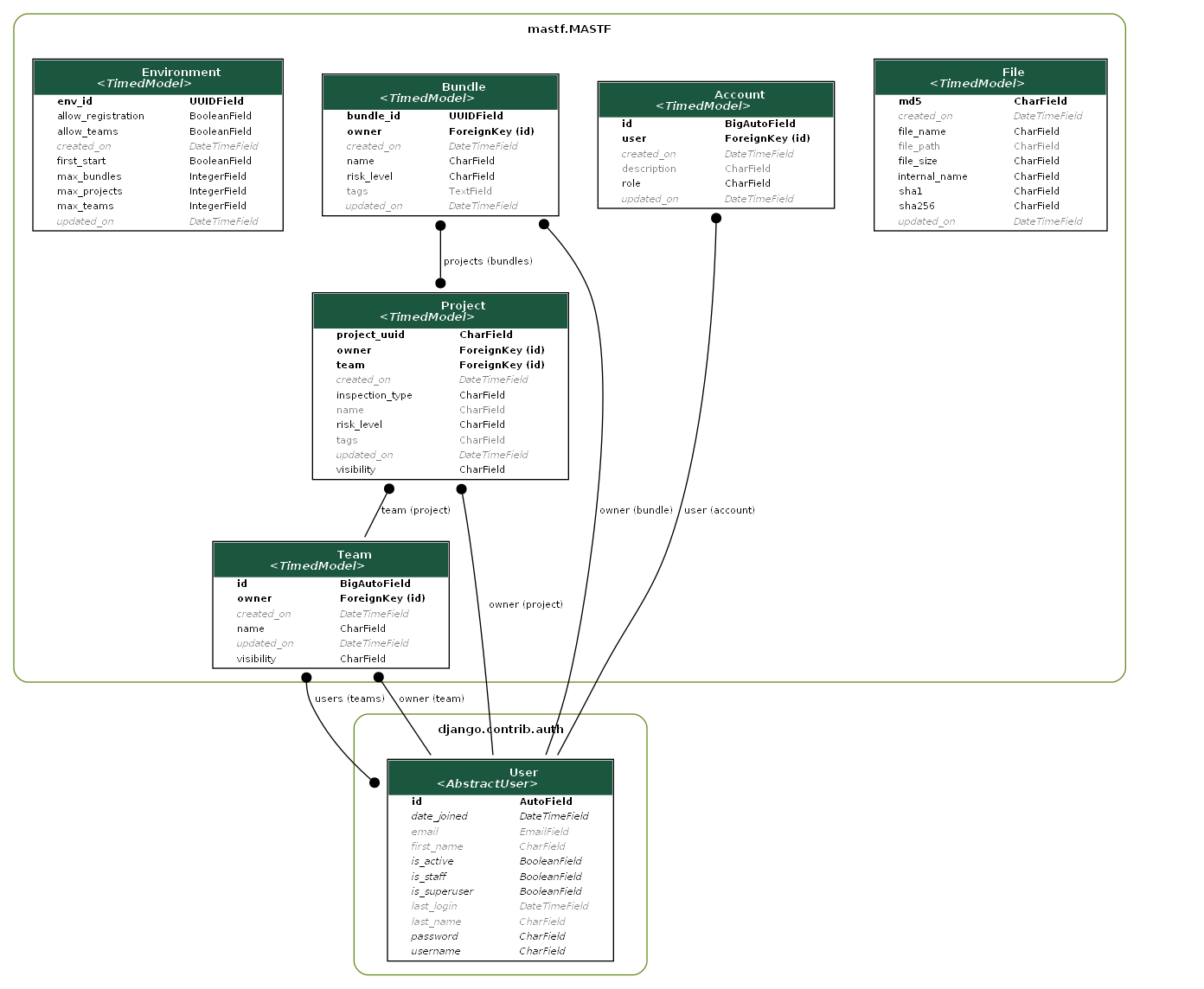
Figure 1: Simple overview of necessary models for a MAST-F instance to work.
- class mastf.MASTF.models.Environment(*args, **kwargs)[source]
The
Environment
class is a Django model representing the configuration of the application’s environment.- allow_registration
A boolean attribute that determines whether new user registration is allowed in the current environment. The default value is
True
.
- allow_teams
A boolean attribute that determines whether teams are allowed in the environment. The default value is
True
.
- static env() Environment [source]
A static method that returns the environment instance.
If an instance is not found, a new one is created with a unique UUID. This method returns the only instance of the Environment model.
- Returns:
the current environment instance
- Return type:
Environment
- env_id
A UUIDField attribute that serves as the primary key of the model. It uniquely identifies the environment instance.
- first_start
Indicates whether the application is starting up for the first time.
- max_bundles
Defines the maximum number of bundles allowed in the environment.
- max_projects
Specifies the maximum number of projects allowed in the environment.
- max_teams
Defines the maximum number of teams allowed in the environment
- class mastf.MASTF.models.Team(*args, **kwargs)[source]
A team contains a set of users.
Note
We rather use ‘Team’ as class name than ‘Group’ as a group class is already defined by Django. Each team can have a list of users.
Serializer Class
TeamSerializer
Form Class
TeamForm
REST Views
TeamView
,TeamListView
andTeamCreationView
- static get(owner: User, name: str) Team [source]
Returns the first team that stores the given name of the provided user.
- Parameters:
owner (User) – the owner or member of a team
name (str) – the team’s name
- Returns:
the first occurrence or None
- Return type:
- static get_by_owner(owner: User, queryset=None) QuerySet [source]
Returns all teams in the scope of that user.
- Parameters:
owner (User) – the team owner or member
queryset (QuerySet, optional) – üre-defined collection of teams, defaults to None
- Returns:
all teams the given user is a member or the owner of
- Return type:
models.QuerySet
- name
The team’s name
- owner
The owner of this team (the user that created the team)
- users
A
many-to-many
relation that simulates a membership in a team
- visibility
The team’s visibility.
Note that internal should not be applied to teams as this state is related to projects.
- class mastf.MASTF.models.Project(*args, **kwargs)[source]
Database model for mobile application projects.
Note
Projects may be assigned to whole teams instead of set only one user in charge of it. All users that are a member of the project’s team are able to modify the project.
This model class also defines utility methods that can be used to retrieve the local system path of the project directory as well as general stats that will be mapped in a
namespace
object.- dir(path: str, create: bool = True) Path [source]
Returns the directory at the specified location.
- Parameters:
path (str) – the local directory name
create (bool, optional) – whether the directory should be created, defaults to True
- Returns:
the created path instance
- Return type:
pathlib.Path
- property directory: Path
Returns the project’s directory.
- Returns:
the directory as
Path
object.- Return type:
pathlib.Path
- static get_by_user(owner: User, queryset: QuerySet = None) QuerySet [source]
Returns all projects where the provided user has access to.
This query attempts to collect all projects that can be modified by the given owner. That includes projects that are assigned to a team of which the provided user is a member; projects that are public and projects that are maintained by the given owner.
Note
Only projects that are globally PUBLIC and not assigned to any team will be included in this list.
- Parameters:
owner (User) – the owner or member
queryset (models.QuerySet, optional) – pre-defined project collection, defaults to None
- Returns:
all projects that fit the requirements
- Return type:
models.QuerySet
- inspection_type
Specifies the inspection type to apply when scanning an app.
- name
Stores the display name of this application.
- owner
Specifies the onwer of this project.
This field my be null as projects can be assigned to whole teams.
- project_uuid
Stores the UUID of this project.
- risk_level
Stores the current risk level (bound to severity types)
- static stats(owner: User) namespace [source]
Collects information about a project a user can edit.
- Parameters:
owner (User) – the project owner
- Returns:
a dictionary covering general stats (count, risk levels)
- Return type:
- tags
Stores tags for this project (comma-spearated)
- team
Specifies the owner of this project. (may be null)
- visibility
Stores the visibility of this project.
- class mastf.MASTF.models.File(*args, **kwargs)[source]
Stores information about the uploaded file.
Note that this model will store information about the uploaded files only; extracted files will be ignored. Each time a scan file is uploaded, it will be saved in the projects directory
- file_name
The file name of the uploaded file.
- file_path
Stores the internal file path. (will be localized separately)
- file_size
The available disk space needed to save the uploaded file
- internal_name
Specifies the uploaded file name.
- md5
The identifier for each app (MD5 of uploaded file)
- sha1
Additional hash value
- sha256
Additional hash value
- class mastf.MASTF.models.Account(*args, **kwargs)[source]
Represents an account associated with a user in the system.
- Parameters:
user (User) – ForeignKey to the User model representing the user that owns this account.
role (str) – The role assigned to this account. One of the choices available in the Role enumeration. Defaults to Role.REGULAR.
description (str | None) – Optional description for this account.
- class mastf.MASTF.models.Bundle(*args, **kwargs)[source]
The
Bundle
class represents a collection of projects that belong to a single owner. Each bundle can be assigned a risk level and can have multiple tags to help with organization.- bundle_id
A UUID field that serves as the primary key for a bundle instance.
- static get_by_owner(owner: User, queryset: QuerySet = None) QuerySet [source]
This method takes a User instance and an optional QuerySet instance and returns a QuerySet containing all bundles that can be modified by the provided owner. This includes bundles that are assigned to a team of which the provided user is a member, public bundles, and bundles maintained by the provided owner. If a QuerySet instance is provided, the method will apply the filter to that queryset.
- Parameters:
owner (User) – the bundle owner
queryset (models.QuerySet, optional) – the initial queryset to use, defaults to None
- Returns:
the filtered queryset
- Return type:
models.QuerySet
- name
A string field that stores the name of the bundle. It has a maximum length of 256 characters and is required.
- owner
A foreign key to the User model that represents the owner of the bundle.
- projects
A many-to-many field that connects the bundle to the
Project
model and allows multiple projects to be associated with a single bundle.
- risk_level
A string field that stores the risk level associated with the bundle. It has a default value of
Severity.NONE
.
- static stats(owner: User) namespace [source]
This method takes a User instance and returns a namespace object that contains statistics about the bundles owned by that user. The namespace object contains the following fields:
count
: The total number of bundles owned by the user.risk_high
: The number of bundles with a risk level of Severity.HIGH.risk_medium
: The number of bundles with a risk level of Severity.MEDIUM.ids
: A set of UUIDs representing the IDs of all the bundles owned by the user.
- Parameters:
owner (User) – the owner
- Returns:
a namespace containing stats about the queried bundles.
- Return type:
- tags
A text field that stores tags associated with the bundle. It is optional and can be left blank.